How To Get Twitch Notifications
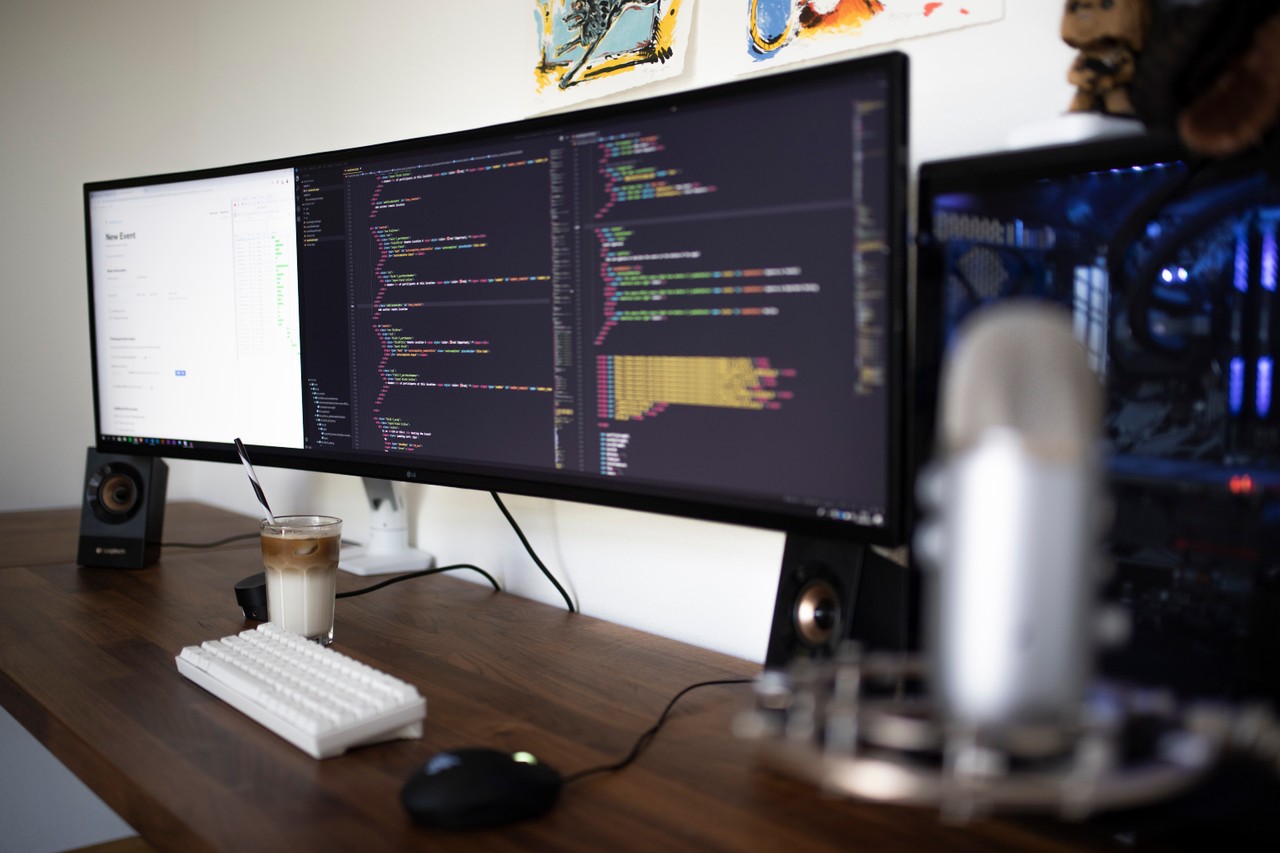
Hi everyone :) Today I am starting time a new series of posts specifically aimed at Python beginners. The concept is rather simple: I'll do a fun project, in as few lines of code as possible, and will effort out as many new tools as possible.
For instance, today we will larn to utilise the Twilio API, the Twitch API, and we'll see how to deploy the project on Heroku. I'll prove yous how you tin can accept your own "Twitch Alive" SMS notifier, in 30 lines of codes, and for 12 cents a month.
Prerequisite: Y'all only demand to know how to run Python on your auto and some bones commands in git (commit & push). If you need assistance with these, I can recommend these 2 articles to you:
Python 3 Installation & Setup Guide
The Ultimate Git Command Tutorial for Beginners from Adrian Hajdin.
What you'll learn:
- Twitch API
- Twilio API
- Deploying on Heroku
- Setting up a scheduler on Heroku
What y'all will build:
The specifications are simple: nosotros want to receive an SMS equally presently as a specific Twitcher is live streaming. We want to know when this person is going alive and when they leave streaming. We want this whole thing to run by itself, all day long.
We will dissever the project into 3 parts. First, nosotros will come across how to programmatically know if a particular Twitcher is online. Then nosotros will see how to receive an SMS when this happens. We volition terminate by seeing how to make this piece of code run every X minutes, so we never miss another moment of our favorite streamer'south life.
Is this Twitcher live?
To know if a Twitcher is live, we tin can do two things: we can go to the Twitcher URL and endeavour to see if the badge "Alive" is there.
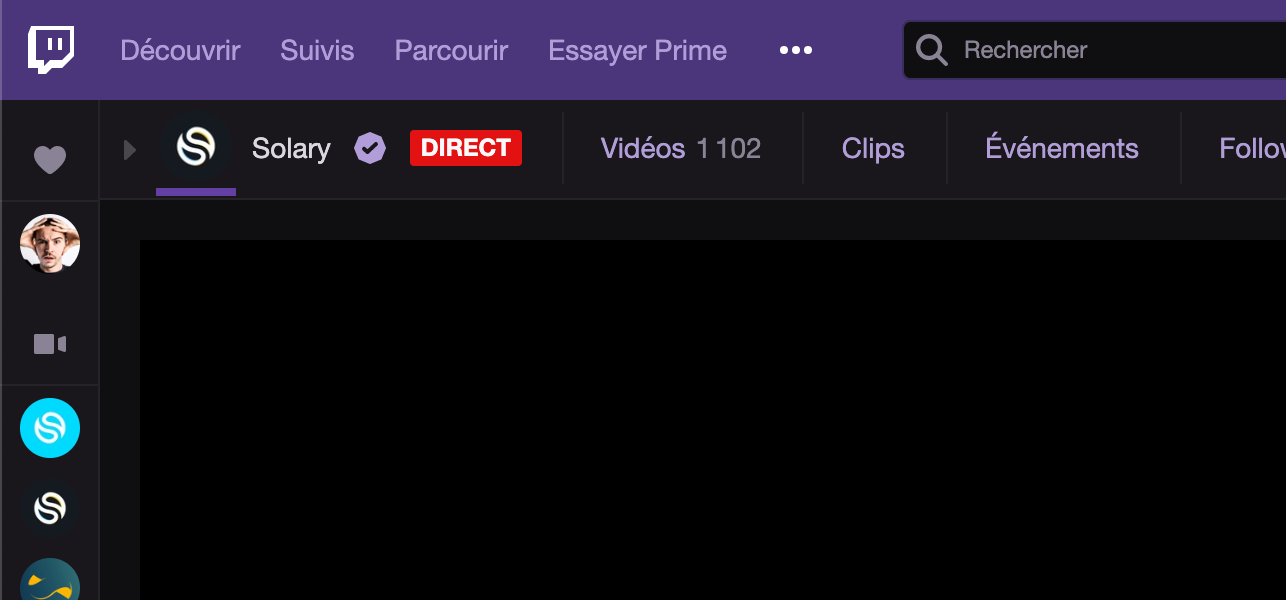
This process involves scraping and is not easily doable in Python in less than xx or and then lines of code. Twitch runs a lot of JS code and a simple asking.get() won't be enough.
For scraping to work, in this example, nosotros would demand to scrape this folio within Chrome to get the same content like what yous see in the screenshot. This is achievable, but it will take much more than 30 lines of code. If y'all'd like to learn more than, don't hesitate to bank check my recent web scraping without getting blocked guide. (I recently launch ScrapingBee, a spider web-scraping tool hence my knowledge in the field ;))
So instead of trying to scrape Twitch, nosotros will use their API. For those unfamiliar with the term, an API is a programmatic interface that allows websites to betrayal their features and data to anyone, mainly developers. In Twitch's instance, their API is exposed through HTTP, witch means that we can have lots of data and do lots of things by just making a simple HTTP asking.
Get your API key
To do this, you accept to beginning create a Twitch API key. Many services enforce authentication for their APIs to ensure that no one abuses them or to restrict access to certain features by certain people.
Please follow these steps to go your API key:
- Create a Twitch account
- Now create a Twitch dev business relationship -> "Signing up with Twitch" top correct
- Get to your "dashboard" once logged in
- "Annals your awarding"
- Proper noun -> Whatsoever, Oauth redirection URL -> http://localhost, Category -> Whatsoever
You should at present see, at the bottom of your screen, your client-id. Go along this for later.
Is that Twitcher streaming now?
With your API key in mitt, we tin now query the Twitch API to take the information we want, so allow'south begin to code. The following snippet just consumes the Twitch API with the correct parameters and prints the response.
# requests is the go to package in python to make http asking # https://ii.python-requests.org/en/master/ import requests # This is one of the route where Twich expose data, # They have many more: https://dev.twitch.television receiver/docs endpoint = "https://api.twitch.tv/helix/streams?" # In order to cosign we need to pass our api key through header headers = {"Customer-ID": "<YOUR-CLIENT-ID>"} # The previously set endpoint needs some parameter, here, the Twitcher we desire to follow # Disclaimer, I don't even know who this is, only he was the first i on Twich to have a live stream so I could accept nice examples params = {"user_login": "Solary"} # It is now time to make the actual request response = request.get(endpoint, params=params, headers=headers) impress(response.json())
The output should look like this:
{ 'data':[ { 'id':'35289543872', 'user_id':'174955366', 'user_name':'Solary', 'game_id':'21779', 'type':'live', 'title':"Wakz duoQ w/ Tioo - GM 400LP - On récupère le chall après les -250LP d'inactivité !", 'viewer_count':4073, 'started_at':'2019-08-14T07:01:59Z', 'language':'fr', 'thumbnail_url':'https://static-cdn.jtvnw.net/previews-ttv/live_user_solary-{width}x{height}.jpg', 'tag_ids':[ '6f655045-9989-4ef7-8f85-1edcec42d648' ] } ], 'pagination':{ 'cursor':'eyJiIjpudWxsLCJhIjp7Ik9mZnNldCI6MX19' } }
This data format is called JSON and is hands readable. The data
object is an array that contains all the currently active streams. The key type
ensures that the stream is currently alive
. This key volition be empty otherwise (in case of an fault, for example).
So if we want to create a boolean variable in Python that stores whether the current user is streaming, all we have to append to our lawmaking is:
json_response = response.json() # We get only streams streams = json_response.become('data', []) # We create a small function, (a lambda), that tests if a stream is alive or not is_active = lambda stream: stream.get('type') == 'live' # We filter our array of streams with this function so we only proceed streams that are agile streams_active = filter(is_active, streams) # any returns Truthful if streams_active has at least one element, else Imitation at_least_one_stream_active = any(streams_active) print(at_least_one_stream_active)
At this betoken, at_least_one_stream_active
is Truthful when your favourite Twitcher is live.
Let's now see how to become notified past SMS.
Ship me a text, At present!
And so to send a text to ourselves, nosotros will use the Twilio API. Just become over there and create an business relationship. When asked to confirm your phone number, please use the telephone number you want to employ in this project. This style you'll be able to use the $15 of free credit Twilio offers to new users. At around 1 cent a text, it should be enough for your bot to run for 1 year.
If you go along the console, you'll see your Business relationship SID
and your Auth Token
, save them for later. Also click on the large red button "Go My Trial Number", follow the step, and salve this one for after too.
Sending a text with the Twilio Python API is very like shooting fish in a barrel, every bit they provide a bundle that does the annoying stuff for you. Install the bundle with pip install Twilio
and merely do:
from twilio.residue import Customer client = Client(<Your Account SID>, <Your Auth Token>) customer.letters.create( body='Examination MSG',from_=<Your Trial Number>,to=<Your Existent Number>)
And that is all you need to send yourself a text, amazing correct?
Putting everything together
Nosotros will at present put everything together, and shorten the code a bit and then we manage to say under 30 lines of Python lawmaking.
import requests from twilio.rest import Customer endpoint = "https://api.twitch.tv/helix/streams?" headers = {"Client-ID": "<YOUR-Customer-ID>"} params = {"user_login": "Solary"} response = request.become(endpoint, params=params, headers=headers) json_response = response.json() streams = json_response.become('data', []) is_active = lambda stream:stream.go('blazon') == 'live' streams_active = filter(is_active, streams) at_least_one_stream_active = whatever(streams_active) if at_least_one_stream_active: customer = Client(<Your Account SID>, <Your Auth Token>) client.messages.create(body='Live !!!',from_=<Your Trial Number>,to=<Your Existent Number>)
Fugitive double notifications
This snippet works slap-up, only should that snippet run every minute on a server, as soon as our favorite Twitcher goes live we will receive an SMS every minute.
Nosotros need a way to store the fact that we were already notified that our Twitcher is live and that we don't need to be notified anymore.
The expert matter with the Twilio API is that it offers a fashion to recall our message history, so nosotros just have to retrieve the concluding SMS we sent to meet if nosotros already sent a text notifying u.s. that the twitcher is live.
Hither what we are going practice to in pseudocode:
if favorite_twitcher_live and last_sent_sms is not live_notification: send_live_notification() if not favorite_twitcher_live and last_sent_sms is live_notification: send_live_is_over_notification()
This style nosotros volition receive a text equally presently as the stream starts, too as when it is over. This way we won't become spammed - perfect right? Permit's lawmaking it:
# reusing our Twilio client last_messages_sent = customer.messages.listing(limit=1) last_message_id = last_messages_sent[0].sid last_message_data = client.messages(last_message_id).fetch() last_message_content = last_message_data.body
Let's now put everything together again:
import requests from twilio.rest import Customer client = Client(<Your Account SID>, <Your Auth Token>) endpoint = "https://api.twitch.idiot box/helix/streams?" headers = {"Client-ID": "<YOUR-Customer-ID>"} params = {"user_login": "Solary"} response = request.get(endpoint, params=params, headers=headers) json_response = response.json() streams = json_response.go('data', []) is_active = lambda stream:stream.become('type') == 'live' streams_active = filter(is_active, streams) at_least_one_stream_active = any(streams_active) last_messages_sent = client.messages.list(limit=1) if last_messages_sent: last_message_id = last_messages_sent[0].sid last_message_data = client.messages(last_message_id).fetch() last_message_content = last_message_data.body online_notified = "Alive" in last_message_content offline_notified = not online_notified else: online_notified, offline_notified = False, Imitation if at_least_one_stream_active and not online_notified: client.messages.create(body='Alive !!!',from_=<Your Trial Number>,to=<Your Real Number>) if not at_least_one_stream_active and not offline_notified: client.messages.create(trunk='OFFLINE !!!',from_=<Your Trial Number>,to=<Your Existent Number>)
And voilà!
You now have a snippet of lawmaking, in less than xxx lines of Python, that volition send yous a text a soon as your favourite Twitcher goes Online / Offline and without spamming you.
We just now need a way to host and run this snippet every X minutes.
The quest for a host
To host and run this snippet we volition utilise Heroku. Heroku is honestly ane of the easiest means to host an app on the web. The downside is that it is actually expensive compared to other solutions out at that place. Fortunately for us, they take a generous free program that will permit usa to do what we desire for almost naught.
If you don't already, you need to create a Heroku account. You lot also need to download and install the Heroku client.
You now take to move your Python script to its own folder, don't forget to add a requirements.txt
file in information technology. The content of the latter begins:
requests twilio
cd
into this binder and only practise a `heroku create --app <app name>`.
If yous go on your app dashboard you lot'll run across your new app.
We now need to initialize a git repo and button the code on Heroku:
git init heroku git:remote -a <app name> git add . git commit -am 'Deploy quantum script' git button heroku chief
Your app is now on Heroku, only it is not doing anything. Since this little script can't accept HTTP requests, going to <app proper noun>.herokuapp.com
won't exercise annihilation. But that should not be a trouble.
To have this script running 24/7 we need to use a unproblematic Heroku add-on phone call "Heroku Scheduler". To install this add-on, click on the "Configure Add together-ons" push on your app dashboard.

And then, on the search bar, look for Heroku Scheduler:

Click on the result, and click on "Provision"
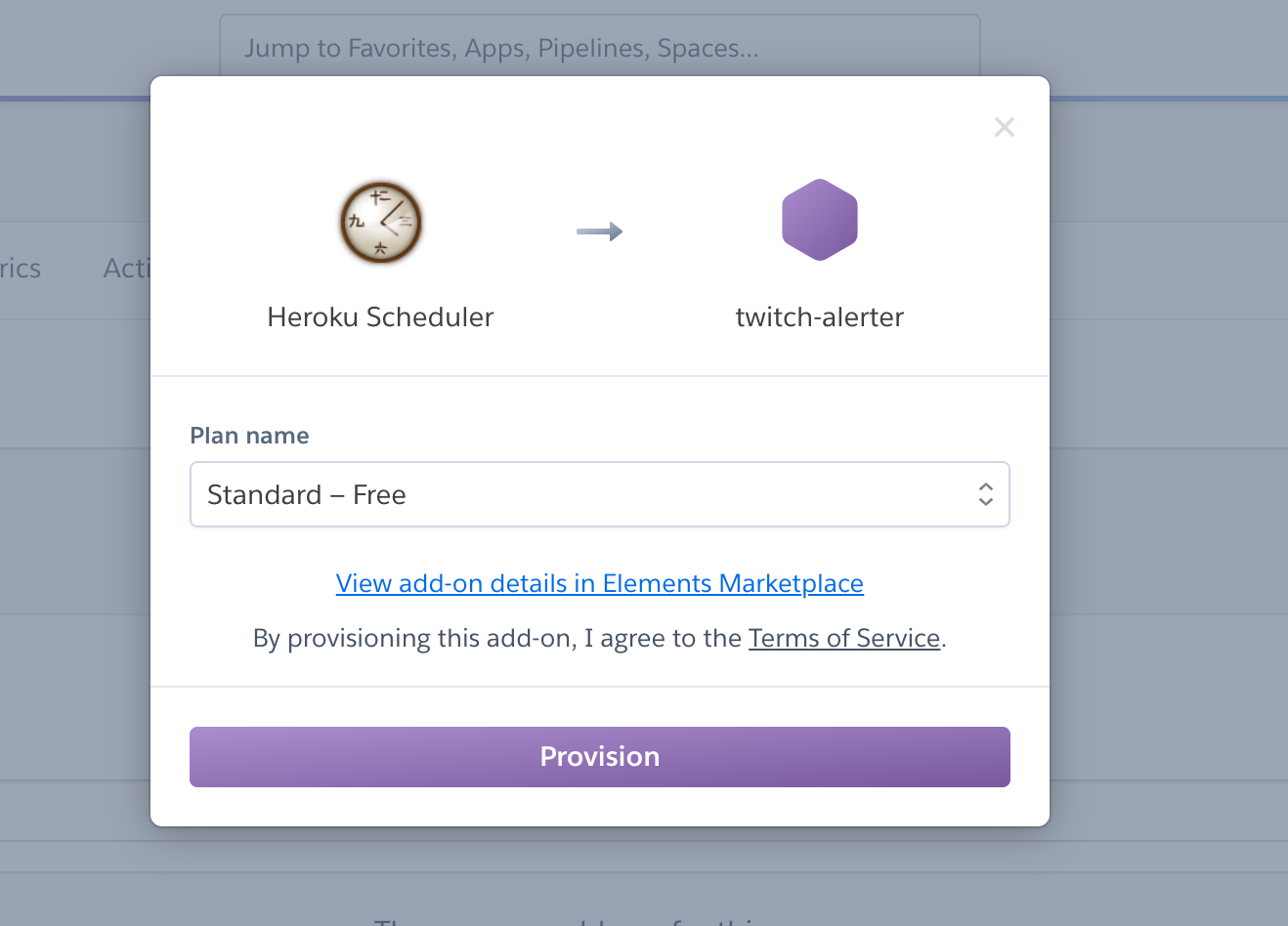
If y'all go back to your App dashboard, you'll run across the add-on:
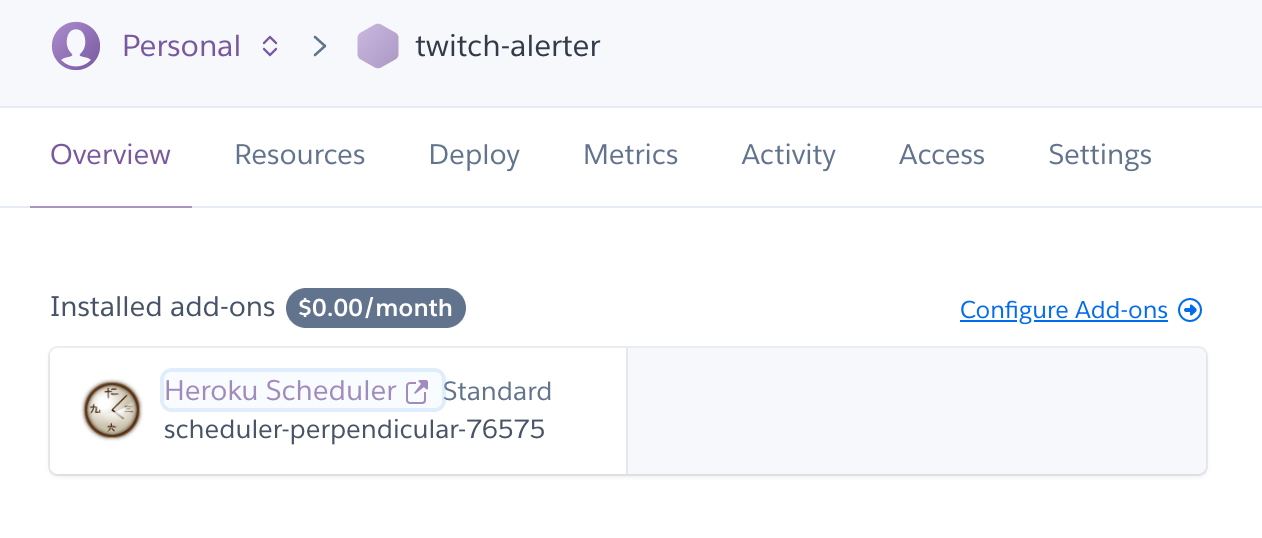
Click on the "Heroku Scheduler" link to configure a job. Then click on "Create Job". Here select "10 minutes", and for run command select `python <name_of_your_script>.py`. Click on "Save job".
While everything we used so far on Heroku is complimentary, the Heroku Scheduler will run the chore on the $25/month instance, only prorated to the 2d. Since this script approximately takes 3 seconds to run, for this script to run every 10 minutes you lot should just accept to spend 12 cents a month.
Ideas for improvements
I promise y'all liked this project and that you had fun putting it into place. In less than thirty lines of code, we did a lot, merely this whole affair is far from perfect. Here are a few ideas to improve it:
- Transport yourself more data about the current streaming (game played, number of viewers ...)
- Ship yourself the duration of the last stream once the twitcher goes offline
- Don't send y'all a text, but rather an email
- Monitor multiple twitchers at the same fourth dimension
Practice not hesitate to tell me in the comments if you have more ideas.
Conclusion
I promise that yous liked this post and that you learned things reading it. I truly believe that this kind of project is one of the best ways to larn new tools and concepts, I recently launched a web scraping API where I learned a lot while making it.
Please tell me in the comments if you liked this format and if you want to do more than.
I have many other ideas, and I hope yous will like them. Practice not hesitate to share what other things you lot build with this snippet, possibilities are endless.
Happy Coding.
Pierre
Don't desire to miss my next mail:
You lot tin can subscribe here to my newsletter.
Learn to code for gratis. freeCodeCamp's open up source curriculum has helped more than xl,000 people get jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/20-lines-of-python-code-get-notified-by-sms-when-your-favorite-team-scores-a-goal/
Posted by: linauntess.blogspot.com
0 Response to "How To Get Twitch Notifications"
Post a Comment